Having received the SPIRIT1 development board (I bought a Nucleo-IDS01A4) I have been attempting to communicate via the SPI interface detailed in the datasheet. As I had a spare RaspberryPi 1 I'm using that as a starting point.
Connecting?
The MBed project has a very useful pinout diagram for the board.
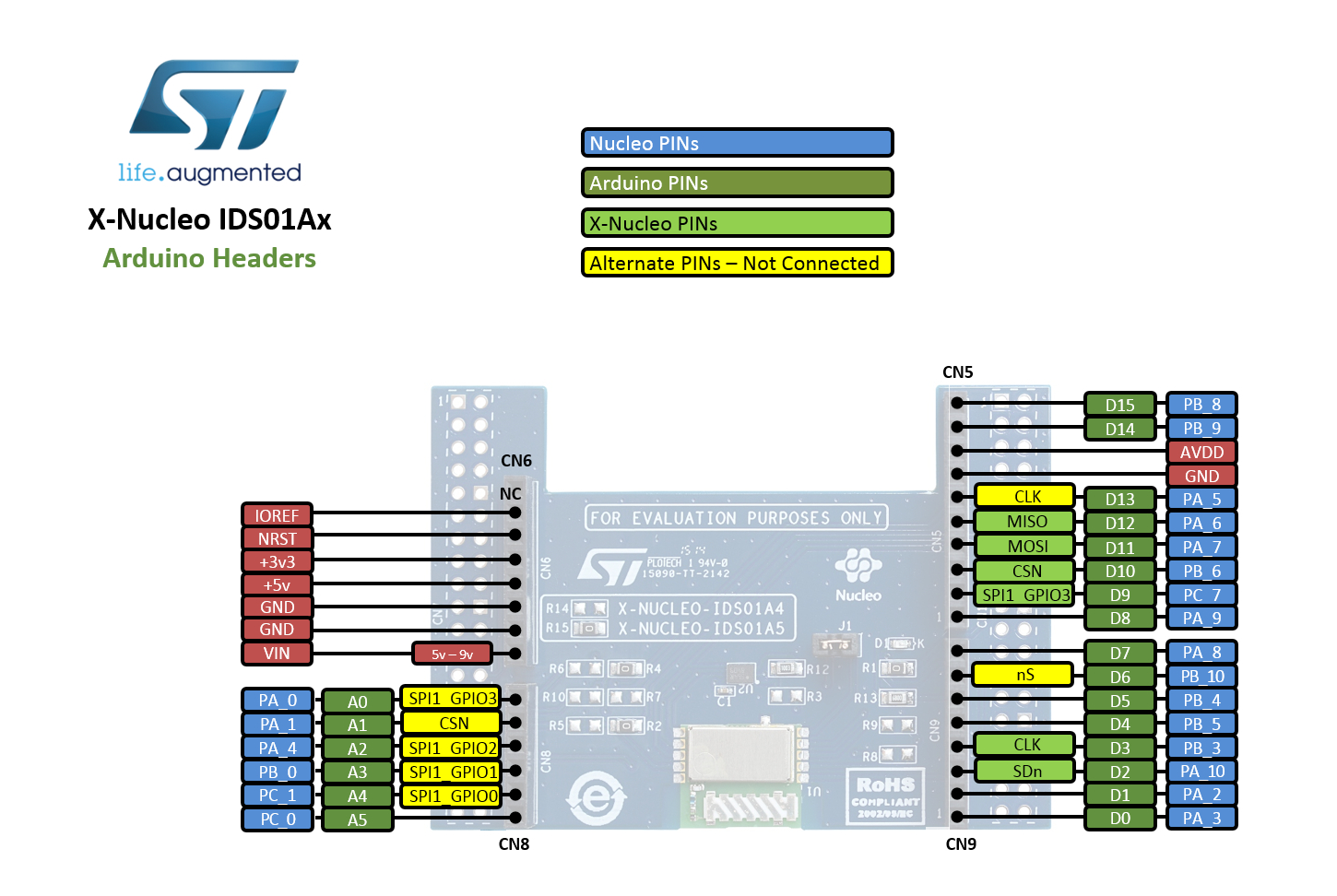
The datasheet helpfully contains details of the SPI interface, so I know it's 4 wire. The board runs at 3.3V and the +3v3 pin is connected via the J1 jumper.
- Pi Pin 1 -> +3v3
- Pi Pin 6 -> GND
- Pi Pin 7 -> SDn
- Pi Pin 19 -> MOSI
- Pi Pin 21 -> MISO
- Pi Pin 23 -> CLK
- Pi Pin 24 -> CSN
If SDn is HIGH then the SPIRIT1 is shutdown, so this pin needs to be pulled LOW before communication. This allows me to have a reset :-)
Serial via Python
Time to fire up some python code...
import spidev
import RPi.GPIO as GPIO
SDn = 7
# Enable the board
GPIO.setmode(GPIO.BOARD)
GPIO.setup(SDn, GPIO.OUT)
GPIO.output(SDn, GPIO.LOW)
# Setup the SPI device using a nice low speed to start
# The mode is as per the SPIRIT1 datasheet.
spirit = spidev.SpiDev()
spirit.open(0, 0)
spirit.max_speed_hz = 250000
spirit.mode = 0b00
# 3 byte command but we expect 4 bytes, so pad with 0x00
received = spirit.xfer2([0x01, 0xF0, 0xF1, 0x00])
print(f"<<< 0x{received[0]:02x}{received[1]:02x}{received[2]:02x}{received[3]:02x}")
This is a very simple start to try and read the DEVICE_INFO[1:0] registers.
$ python3 ./simple.py
<<< 0x52070130
The datasheet shows that the MC_STATE[1:0] will be returned before any requested registers, so my request for 2 registers generates a response of 4 bytes.
MC_STATE - 0x5207
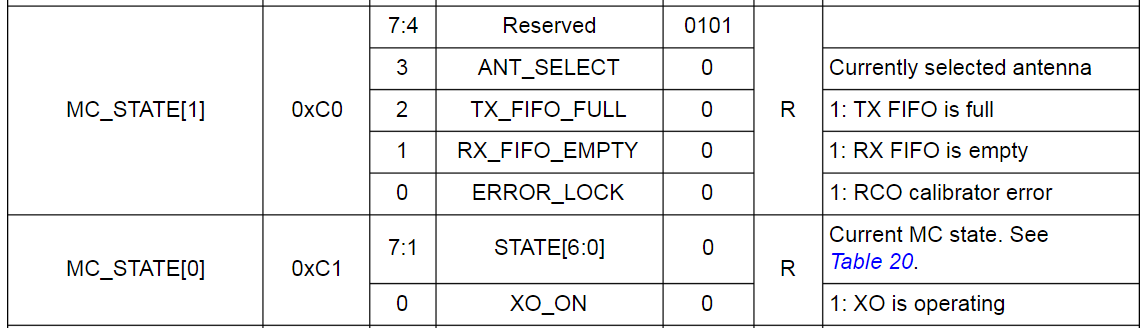
The upper byte is 0x57 -> 0101 0010.
The 4 reserved bits are present, followed by the RX_FIFO_EMPTY flag.
The lower byte, 0x07 contains 7 bits of the current state and a final bit for XO_ON.
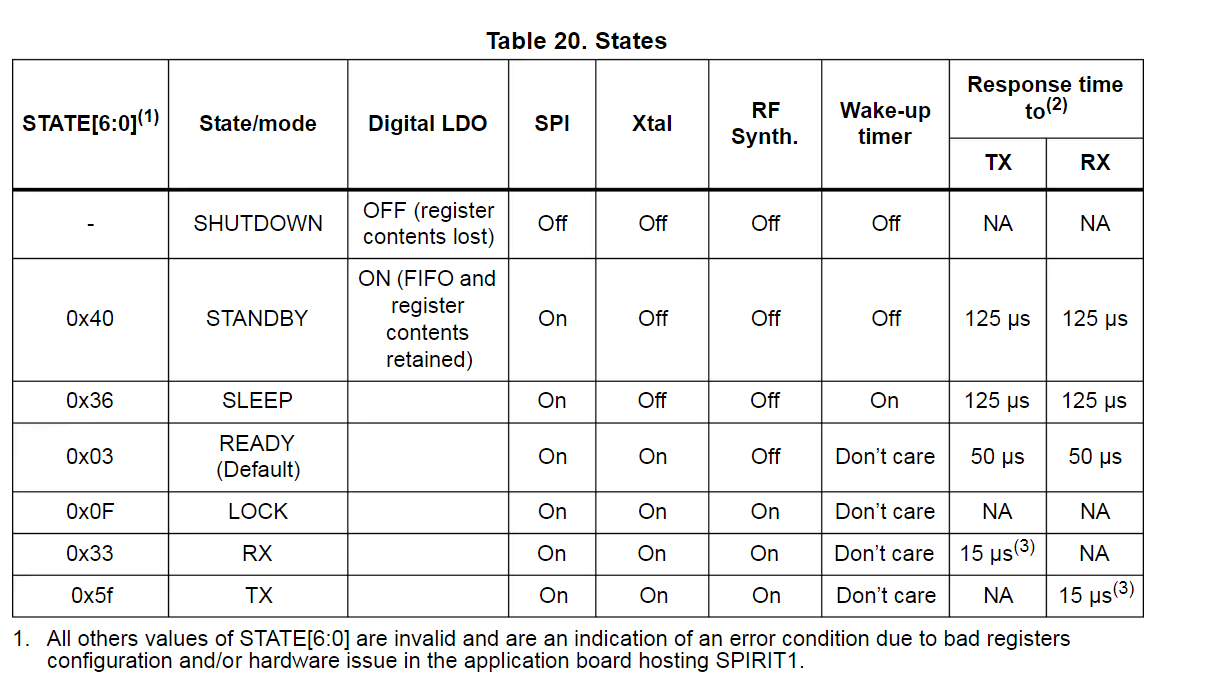
>>> 0x07 >> 1
3
This seems to make sense as we have a STATE of 3 which equates to READY and we have the XO_ON flag set.
DEVICE_INFO - 0x0130

PulseView
While experimenting I hooked up the small logic analyser I have and ran PulseView to observe what was going on. The traces confirmed the above.

This isn't the first time I have tried using the logic analyser, but it's the first time I've had such good results from it. There is no substitute for actually using it to gain experience.
Next Steps
The next step is to start building a small class to allow me to work with the module. I'm using Python as I hope to connect it to Home Assistant at some point.